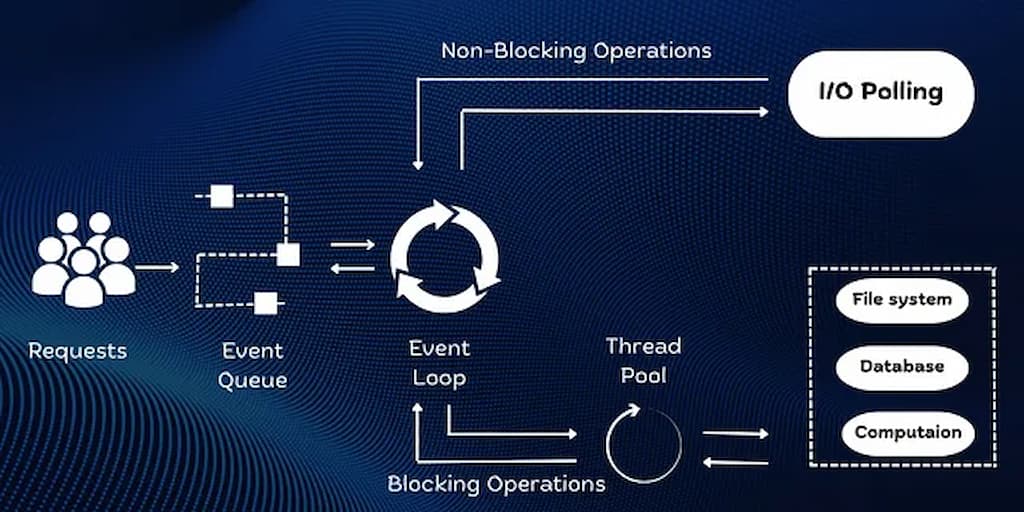
Node.js is renowned for efficiently handling thousands of concurrent connections, despite...
Node.js is renowned for efficiently handling thousands of concurrent connections, despite relying on a single-threaded event loop model. In this article, we'll break down the Node.js event loop, explain how it manages asynchronous tasks, and clarify these concepts with practical examples.
TheEvent Loopis the core mechanism in Node.js that enables it to perform non-blocking I/O operations and handle asynchronous tasks efficiently on a single thread.
At a high level, Node.js offloads time-intensive operations to background threads, and once complete, their callbacks are queued for execution on the main thread.
Node.js uses an event-driven, non-blocking I/O model:
This model prevents blocking the main thread, allowing Node.js to remain highly efficient.
The Event Loop operates in distinct phases:
setTimeout
and setInterval
.setImmediate
callbacks.close
callbacks.┌───────────────────────────┐
│ Timers │
├───────────────────────────┤
│ Pending Callbacks │
├───────────────────────────┤
│ Idle, Prepare │
├───────────────────────────┤
│ Poll │
├───────────────────────────┤
│ Check │
├───────────────────────────┤
│ Close │
└───────────────────────────┘
console.log('Start');
setTimeout(() => {
console.log('Timeout');
}, 0);
setImmediate(() => {
console.log('Immediate');
});
console.log('End');
Start
End
Immediate
Timeout
Start
and End
execute synchronously.setTimeout
schedules a callback in theTimers Phase.setImmediate
schedules a callback in theCheck Phase.setImmediate
executes before setTimeout
because theCheck Phaseoccurs before the nextTimers Phase.const fs = require('fs');
console.log('Start');
fs.readFile('./file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log('File Read');
});
setImmediate(() => {
console.log('Immediate');
});
console.log('End');
Start
End
Immediate
File Read
Start
and End
execute synchronously.fs.readFile
operates asynchronously in thePoll Phase.setImmediate
executes during theCheck Phasebefore the file read callback.setImmediate
for tasks that should execute after the current phase.node --inspect
.The Node.js Event Loop is the backbone of its non-blocking architecture, enabling efficient concurrency and resource management. By understanding its phases and behavior, developers can build performant and scalable applications.
Mastering the Event Loop isn't just about knowing the phases; it's about leveraging this knowledge to write efficient, bug-free code.