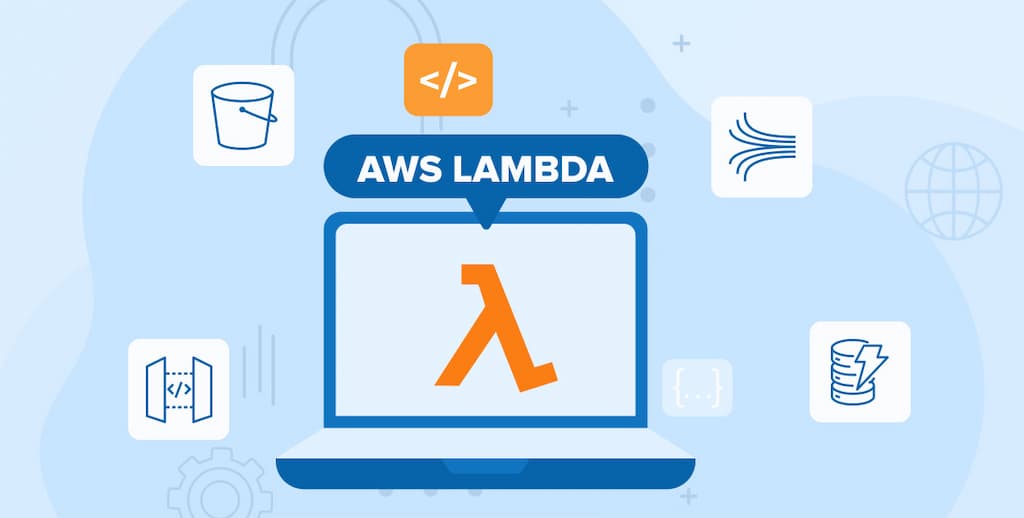
What is AWS Lambda? AWS Lambda is a serverless computing service offered by Amazon Web...
AWS Lambda is a serverless computing service offered by Amazon Web Services (AWS) that enables users to run code without the burden of server provisioning and management. Essentially, it's a Function-as-a-Service (FaaS) platform where you only focus on writing your code, and AWS takes care of everything else related to running and scaling it. Lambda functions are triggered by events, which could be anything from a file upload to an API request.
AWS Lambda works on an event-driven architecture. You upload your code, and when a specific event occurs that triggers your function, Lambda automatically provisions the necessary compute resources, executes your code within a container, and manages its entire lifecycle.
Here's a step-by-step breakdown of the process:
Event Trigger:An event occurs, such as an HTTP request to an API Gateway endpoint or a file upload to an S3 bucket.
Lambda Invocation:AWS Lambda receives the event and triggers the corresponding Lambda function.
Container Creation:Lambda creates a new container for the function, isolating it from other functions.
Code Execution:Your code, packaged as a ZIP file or a container image, is loaded and executed within the container.
Resource Allocation:The container is allocated the necessary resources, like RAM and CPU, as defined in your function configuration.
Output Generation:The function executes your code and generates an output, which could be a response to the API request, a modification to data, or a trigger for another AWS service.
Container Teardown:After execution, the container is torn down, and resources are released.
Billing:You are billed based on the function's execution time and the allocated memory.
Key Points:
AWS Lambda offers several compelling features that make it a popular choice for serverless computing:
1. Cost Savings with Pay-as-you-go model:
2. Event-driven Architecture:
3. Scalability and Availability:
4. Supports Multiple Languages and Frameworks:
5. Serverless Execution:
6. Auto Scaling and High Availability:
7. Integrates with other AWS Services:
8. Versioning and Deployment:
9. Security and Identity Management:
Lambda functions are the heart of your serverless application logic. They contain the code that processes events and performs the desired actions. You write this code in one of the supported programming languages and package it as a deployment package, either a ZIP file or a container image.
Key Aspects of Lambda Function Code:
Handler Function:This is the entry point for your Lambda function, defined in your code. When Lambda invokes your function, it calls the handler function, passing event data and context information as parameters.
Event Object:This object contains all the information about the event that triggered the Lambda function, such as the source of the event, relevant data associated with the event, and metadata about the invocation.
Context Object:This object provides information about the invocation, function, and execution environment. It includes details like the function name, request ID, memory limit, and remaining execution time.
Output:Your Lambda function can return a response, which can be used to communicate with other services or provide feedback to the caller.
AWS Lambda is particularly well-suited for building serverless microservice architectures, where applications are decomposed into small, independent services. Here's how Lambda fits into an e-commerce application architecture:
REST API and CRUD endpoints:Lambda functions, coupled with API Gateway, can create RESTful APIs to handle requests from clients. They can manage CRUD operations (Create, Read, Update, Delete) on product catalogs, shopping carts, orders, and other data.
Data persistence:DynamoDB, a serverless NoSQL database, can be used for data storage and retrieval. Lambda functions can interact with DynamoDB to perform operations like adding items to a shopping cart, retrieving product information, or updating order statuses.
Decoupling microservices with events:EventBridge allows for asynchronous communication between microservices through events. For example, a Lambda function processing a new order can emit an event that triggers other functions to handle inventory updates, payment processing, and shipping notifications.
Message Queues:SQS provides a message queue for asynchronous communication between services. Lambda functions can be triggered by messages in an SQS queue, enabling reliable processing of tasks that might take longer or require retries.
Cloud stack development with IaC:Infrastructure-as-code tools like AWS CDK can automate the deployment of Lambda functions, API Gateway configurations, DynamoDB tables, and other resources, streamlining the development and management of your serverless infrastructure.
Triggers are the mechanisms that initiate the execution of a Lambda function. Lambda supports a wide array of triggers, allowing functions to respond to various events.
Common Triggers:
The versatility of AWS Lambda makes it suitable for a wide range of use cases:
Lambda functions are executed on demand in response to events, following a specific lifecycle managed by the AWS Lambda service.
Steps in Lambda Function Execution:
The Lambda Handler is the entry point for your function's code, acting as the interface between the Lambda runtime and your code. It's a function that receives the event data and context object as input, processes the information according to your logic, and produces an output.
Key Roles of the Lambda Handler:
When you click here , the AWS Management Console will open in a new browser window, so you can keep this step-by-step guide open. In the top navigation bar, search forLambdaand open the AWS Lambda Console.
Blueprints provide example code to do some minimal processing. Most blueprints process events from specific event sources, such as Amazon S3, Amazon DynamoDB, or a custom application.
a. In the AWS Lambda console, chooseCreate function.
Note:The console shows this page only if you do not have any Lambda functions created. If you have created functions already, you will see the Lambda > Functions page. On the list page, choose Create a function to go to theCreate functionpage.
A Lambda function consists of code you provide, associated dependencies, and configuration. The configuration information you provide includes the compute resources you want to allocate (for example, memory), execution timeout, and an IAM role that AWS Lambda can assume to execute your Lambda function on your behalf.
Basic information:
Lambda function code:
In this section, you can review the example code authored in Python.
To continue building your function:
b. Selectuse a blueprint.
c. In theBlueprint namebox, ensure Hello world function with python 3.10 blueprint is selected.
d. In theFuction namebox, enter hello-world-python .
e. ForExecution role, select Create a new role from AWS policy templates.
f. In theRole namebox, enter lambda_basic_execution .
g. Press theCreate Functionbutton.
After creating your function, review other settings.
In this example, Lambda identifies this from the code sample and this should be pre-populated with lambda_function.lambda_handler.
The console shows the hello-world-python Lambda function. You can now test the function, verify results, and review the logs.
a. SelectConfigure Test Eventfrom the drop-down menu calledTest.
b. The editor pops up so you can enter an event to test your function.
Type in an event name like HelloWorldEvent .
Retain default setting of Private forEvent sharing settings.
Choose hello-world from the template list.
You can change the values in the sample JSON, but don’t change the event structure. For this tutorial, replace value1 with hello, world!.
SelectCreate.
c. ChooseTest.
d. Upon successful execution, view the results in the console:
AWS Lambda automatically monitors Lambda functions and reports metrics through Amazon CloudWatch. To help you monitor your code as it executes, Lambda automatically tracks the number of requests, the latency per request, and the number of requests resulting in an error and publishes the associated metrics.
a. Invoke the Lambda function a few more times by repeatedly choosing theTestbutton. This will generate the metrics that can be viewed in the next step.
b. Select theMonitortab to view the results.
c. Scroll down to view the metrics for your Lambda function. Lambda metrics are reported through Amazon CloudWatch. You can leverage these metrics to set custom alarms. For more information about CloudWatch, see the Amazon CloudWatch Developer Guide .
The Monitoring tab will show seven CloudWatch metrics: Invocations, Duration, Error count and success rate (%), Throttles, Async delivery failures, IteratorAge, and Concurrent executions.
With AWS Lambda, you pay for what you use. After you hit your AWS Lambda free tier limit, you are charged based on the number of requests for your functions (invocation count) and the time your code executes (invocation duration). For more information, see AWS Lambda Pricing.
While you will not get charged for keeping your Lambda function, you can easily delete it from the AWS Lambda console.
a. Select theActionsbutton and selectDelete function.
b. You will be asked to confirm your termination - selectDelete.
AWS Lambda is a revolutionary serverless computing service that enables developers to build and run applications without the need for server management. Its event-driven architecture, pay-as-you-go model, seamless integration with other AWS services, and support for various programming languages make it an ideal choice for modern application development. From simple functions to complex microservice architectures, AWS Lambda empowers developers to focus on code and innovation, leaving the complexities of infrastructure management to AWS.