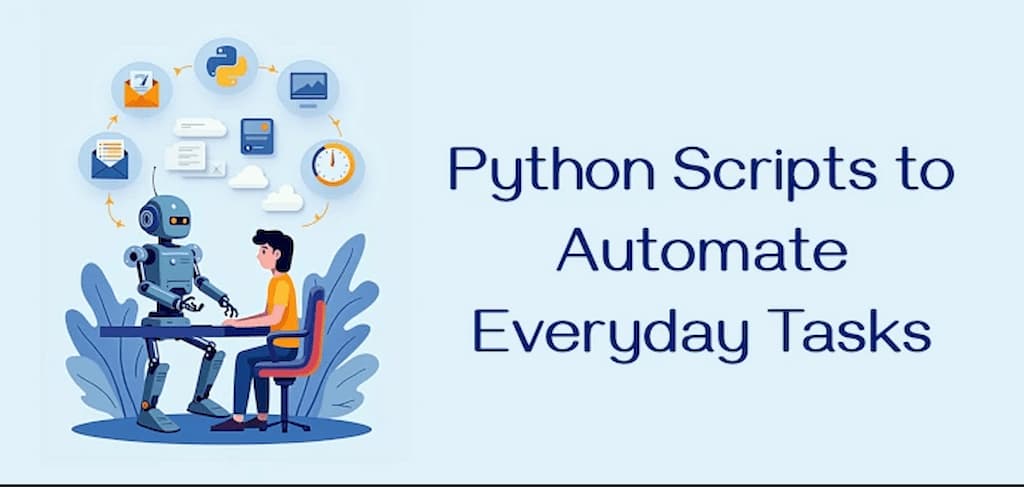
Discover how Python scripting can streamline daily tasks like file organization, web scraping, email automation, and more. Boost productivity with practical examples.
Let’s be real—nobody likes doing repetitive tasks. Whether it's renaming files, scraping data, or sending emails, these tasks drain your time. But what if I told you that Python scripting can handle all of that for you? Imagine writing a script once and letting it do the work forever. That’s the power of automation.
And guess what? Python Developer Resources - Made by 0x3d.site is packed with tools, articles, and trending discussions to help you master Python scripting and automate like a pro.
Let’s break down some real-world scripting examples that will make your life easier.
Is your downloads folder a mess? Python can automatically sort files into folders based on their type.
import os
import shutil
source_folder = "./Downloads"
destination_folders = {
"Images": [".jpg", ".png", ".gif"],
"Documents": [".pdf", ".docx", ".txt"],
"Videos": [".mp4", ".mov", ".avi"],
}
for file in os.listdir(source_folder):
file_path = os.path.join(source_folder, file)
if os.path.isfile(file_path):
for folder, extensions in destination_folders.items():
if any(file.endswith(ext) for ext in extensions):
os.makedirs(os.path.join(source_folder, folder), exist_ok=True)
shutil.move(file_path, os.path.join(source_folder, folder))
Run this script, and your files will be neatly organized into folders!
Need to gather data from a website? Python can do that while you sleep.
import requests
from bs4 import BeautifulSoup
url = "https://example-blog.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
for title in soup.find_all("h2"):
print(title.text)
This script extracts blog titles in seconds. No more manual copying and pasting!
Want to send automatic emails? Python makes it easy.
import smtplib
from email.message import EmailMessage
msg = EmailMessage()
msg.set_content("Hey there! This is an automated email.")
msg["Subject"] = "Python Scripting Automation"
msg["From"] = "your_email@example.com"
msg["To"] = "recipient@example.com"
server = smtplib.SMTP_SSL("smtp.gmail.com", 465)
server.login("your_email@example.com", "your_password")
server.send_message(msg)
server.quit()
Now, you can automate reports, reminders, and notifications!
Spreadsheets taking up your time? Python can update them automatically.
import pandas as pd
data = pd.read_excel("sales.xlsx")
data["Total"] = data["Quantity"] * data["Price"]
data.to_excel("updated_sales.xlsx", index=False)
No more manually calculating totals—Python has your back.
Want your script to run at a specific time? Use Python’s scheduling tools.
import schedule
import time
def morning_task():
print("Good morning! Running your daily automation task...")
schedule.every().day.at("08:00").do(morning_task)
while True:
schedule.run_pending()
time.sleep(60)
Schedule reports, backups, or reminders effortlessly.
The best developers stay updated with new techniques and tools.
Python scripting is your key to working smarter, not harder.
Stop wasting time on manual tasks—start scripting your way to freedom!