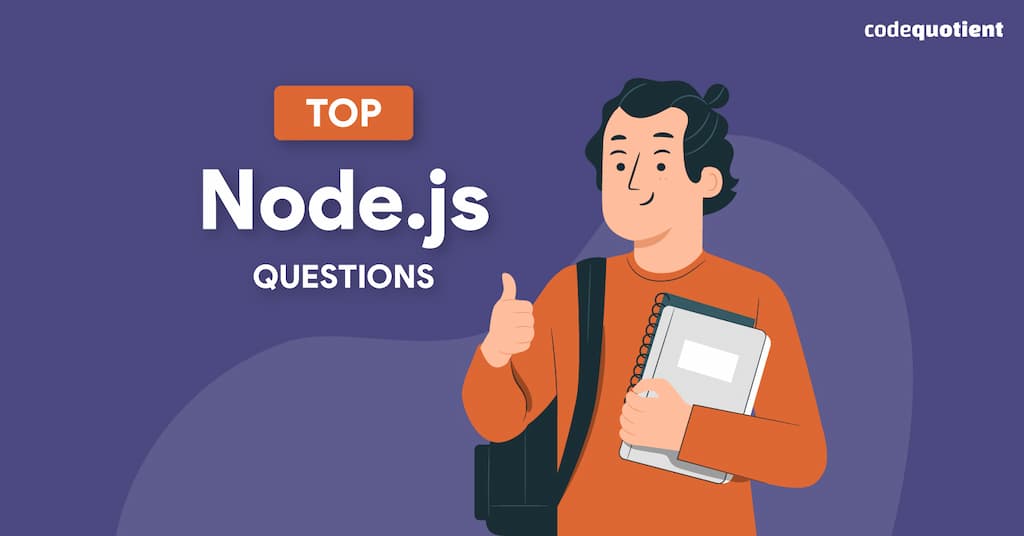
1. What is Node.js? Node.js is an open-source, cross-platform, JavaScript runtime...
Node.js is anopen-source,cross-platform,JavaScript runtime environmentthat executes JavaScript code outside of a web browser. It uses theV8 JavaScript engine, developed by Google, which compiles JavaScript directly into machine code, contributing to its exceptional performance and efficiency. It allows developers to run JavaScript on the server side, enabling the creation of scalable and efficient server-side applications.
Example:
const http = require('http');
http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, World!\n');
}).listen(3000);
console.log('Server running at http://localhost:3000/');
TheEvent Loopis a core concept in Node.js that handles asynchronous operations.
Below is a visual representation of the Event Loop phases to enhance clarity:
setTimeout
and setInterval
callbacks.setImmediate()
callbacks.close
events (e.g., socket.on('close')).Example:
console.log('Start');
setTimeout(() => console.log('Timeout'), 0);
process.nextTick(() => console.log('NextTick'));
console.log('End');
Output:
Start
End
NextTick
Timeout
Comparison with Traditional Web Servers:
Node.js is single-threaded, butclustersallow it to utilize multiple CPU cores.
Example:
const cluster = require('cluster');
const http = require('http');
const os = require('os');
if (cluster.isMaster) {
os.cpus().forEach(() => cluster.fork());
cluster.on('exit', (worker) => {
console.log(`Worker ${worker.process.pid} exited`);
cluster.fork();
});
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end(`Hello from Worker ${process.pid}`);
}).listen(3000);
console.log(`Worker ${process.pid} started`);
}
Example:
/admin
route.Middleware functions are functions executed during the request-response cycle.
req
and res
objects.Example:
app.use((req, res, next) => {
console.log('Middleware executed');
next();
});
Used for interacting with systems and tools without GUI.
Example:
node index.js --port=3000
Example:
try {
throw new Error('Something went wrong');
} catch (err) {
console.error(err.message);
}
Example:
fs.readFile('file.txt', (err, data) => console.log(data));
console.log('This runs first');
Handles uncaught exceptions to prevent application crashes.
Example:
process.on('uncaughtException', (err) => {
console.error('Uncaught Exception:', err);
});
Example Structure:
app/
├── models/
│ └── userModel.js
├── views/
│ └── userView.ejs
├── controllers/
│ └── userController.js
└── routes/
└── userRoutes.js
I/O stands forInput/Outputand refers to any operation that involves reading data from or writing data to an external resource, such as a file, database, or network.
Example:
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Acallbackis a function passed as an argument to another function, which gets executed once the asynchronous task is complete.
Example:
fs.readFile('file.txt', (err, data) => {
if (err) console.error(err);
else console.log(data.toString());
});
This guide provides a professional overview of key Node.js concepts, useful for both interview preparation and real-world applications.