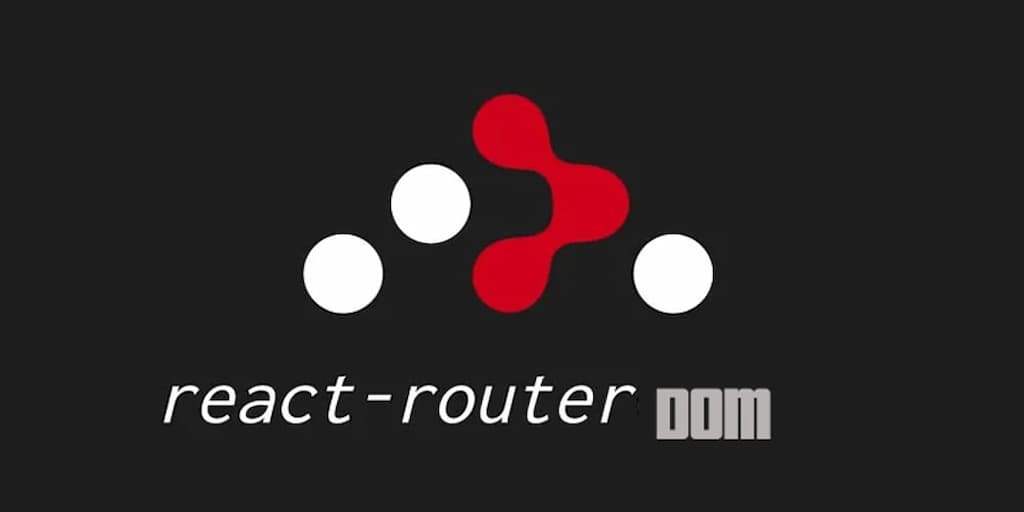
React Router DOM is a powerful library that facilitates seamless client-side routing in React applications
Navigating a single-page application (SPA) can be challenging without a robust routing system. React Router DOM is a powerful library that facilitates seamless client-side routing in React applications. This blog post will walk you through the essential concepts and usage of React Router DOM 6.25.1 to create a dynamic and responsive web application.
First, let's create a Root
component that will serve as the layout for our application, including a header, footer, and an outlet for rendering child components.
import React from "react";
import React from "react";
import { Outlet } from "react-router-dom";
const Root = () => {
return (
<>
<Header />
<Outlet />
<Footer />
</>
);
};
export default Root;
To enable client-side routing, we need to create a Browser Router and configure our routes.
import { createBrowserRouter, RouterProvider } from "react-router-dom";
import ReactDOM from "react-dom";
import Root from './Root';
import Dashboard from './Dashboard';
import About from './About';
import User from './User'
import BigSpinner from './BigSpinner';
const router = createBrowserRouter([
{
path: "/",
element: <Root />,
children: [
{ path: "dashboard", element: <Dashboard /> },
{ path: "about", element: <About /> },
{path="user/:id" element={<User />},
],
},
]);
ReactDOM.createRoot(document.getElementById("root")).render(
<RouterProvider router={router} fallbackElement={<BigSpinner />} />
);
If you are not server-rendering your app, createBrowserRouter
will initiate all matching route loaders when it mounts. During this time, you can provide a fallbackElement
to indicate that the app is working.
<RouterProvider
router={router}
fallbackElement={<SpinnerOfDoom />}
/>
Alternatively, you can use createRoutesFromElements
to define your routes.
import {
createBrowserRouter,
RouterProvider,
createRoutesFromElements,
Route,
} from "react-router-dom";
import Root from './Root';
import Dashboard from './Dashboard';
import About from './About';
const router = createBrowserRouter(
createRoutesFromElements(
<Route path="/" element={<Root />}>
<Route path="dashboard" element={<Dashboard />} />
<Route path="about" element={<About />} />
<Route path="user/:id" element={<User />}
</Route>
)
);
A component allows users to navigate to another page by clicking or tapping on it. It renders an accessible
element with a real
href
.
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/services">Services</Link></li>
<li><Link to="/contact">Contact</Link></li>
<li><Link to="/about">About Us</Link></li>
</ul>
</nav>
A
<navlink></navlink>
is a special kind of <Link>
that knows whether or not it is "active," "pending," or "transitioning."
<Link><ul>
<li>
<NavLink className={({ isActive }) => (isActive ? "active" : "")} to="/">
Home
</NavLink>
</li>
<li>
<NavLink className={({ isActive }) => (isActive ? "active" : "")} to="/services">
Services
</NavLink>
</li>
<li>
<NavLink to="/contact">Contact</NavLink>
</li>
<li>
<NavLink to="/about">About Us</NavLink>
</li>
</ul>
An <outlet></outlet>
renders the child route elements. It is used in parent route components to display nested routes.
useNavigate
The useNavigate
hook provides a way to navigate programmatically.
import { useNavigate } from 'react-router-dom';
function Home() {
const navigate = useNavigate();
const goToAbout = () => {
navigate('/about');
};
return (
<div>
<h1>Home Page</h1>
<button onClick={goToAbout}>Go to About</button>
</div>
);
}
useParams
The useParams
hook returns an object of key/value pairs of the dynamic parameters from the current URL.
import { useParams } from 'react-router-dom';
function UserProfile() {
const { userId } = useParams();
return (
<div>
<h1>User Profile for User ID: {userId}</h1>
</div>
);
}
React Router DOM supports nested routes, allowing you to define routes within other routes.
<Route path="project" element={<Projects />}>
<Route index element={<h1>Feature Project</h1>} />
<Route path="all" element={<h1>All Projects</h1>} />
<Route path="feature" element={<h1>Feature Project</h1>} />
<Route path="new" element={<h1>New Project</h1>} />
</Route>
In this example, the index
route specifies the default content to render when /project
is accessed.
React Router DOM 6.25.1 simplifies the process of client-side routing in React applications. With features like programmatic navigation, nested routes, and hooks like useNavigate
and useParams
, you can build dynamic and responsive web applications with ease. Whether you're developing a small app or a large-scale project, React Router DOM provides the tools you need to manage routing effectively.