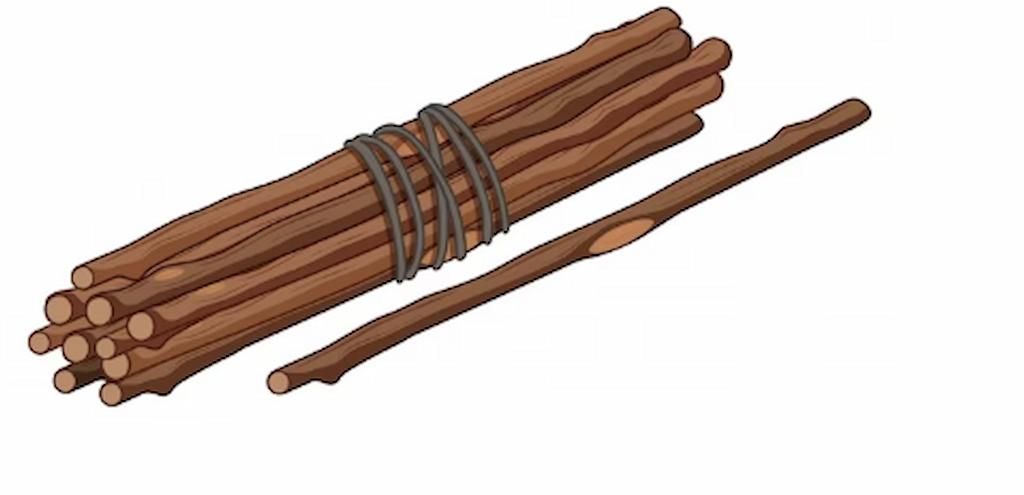
Overview of how to use esbuild to bundle a basic React application.
Bundling is an important phase in the web development process, particularly when dealing with JavaScript frameworks like React. It entails combining all the various JavaScript files and dependencies into a single file for faster browser loading and execution.
esbuild is a lightweight and efficient bundler for React apps.
We'll start by installing esbuild globally in your system by running npm install -g esbuild
in the command line. This will install the latest version of esbuild globally on your system.
After installation, you can access esbuild from the command line by typing esbuild
.
To create a new directory for your React application, open the terminal and navigate to the directory where you want to create the new directory. Then run the following command:
mkdir my-react-app
This will create a new directory named my-react-app
. You can replace it with whatever name you want to give your React application directory.
After creating the directory, navigate into it by running:
cd my-react-app
Initialize a new npm
project by running the following command and following the prompts:
npm init -y
Install React and React DOM by running npm install react react-dom
in the terminal. This will install the latest versions of React and React DOM in your project, along with any required dependencies.
Let's create the necessary files and folders. Here's a basic structure:
my-react-app
├── src
| |
│ |── index.js
├── |--- index.html
│
└── package.json
Add the code shown below in your app, following the above structure:
index.html:
Hello, esbuild!
The above code outlines the fundamental structure of an HTML5 web page. It starts with a declaration indicating the use of HTML5. The main structure consists of an
root element containing asection for metadata, including character encoding and viewport settings for responsiveness.
The Additionally, there's a
element defines the browser tab's title, while the actual content resides in theelement. Within the
, a