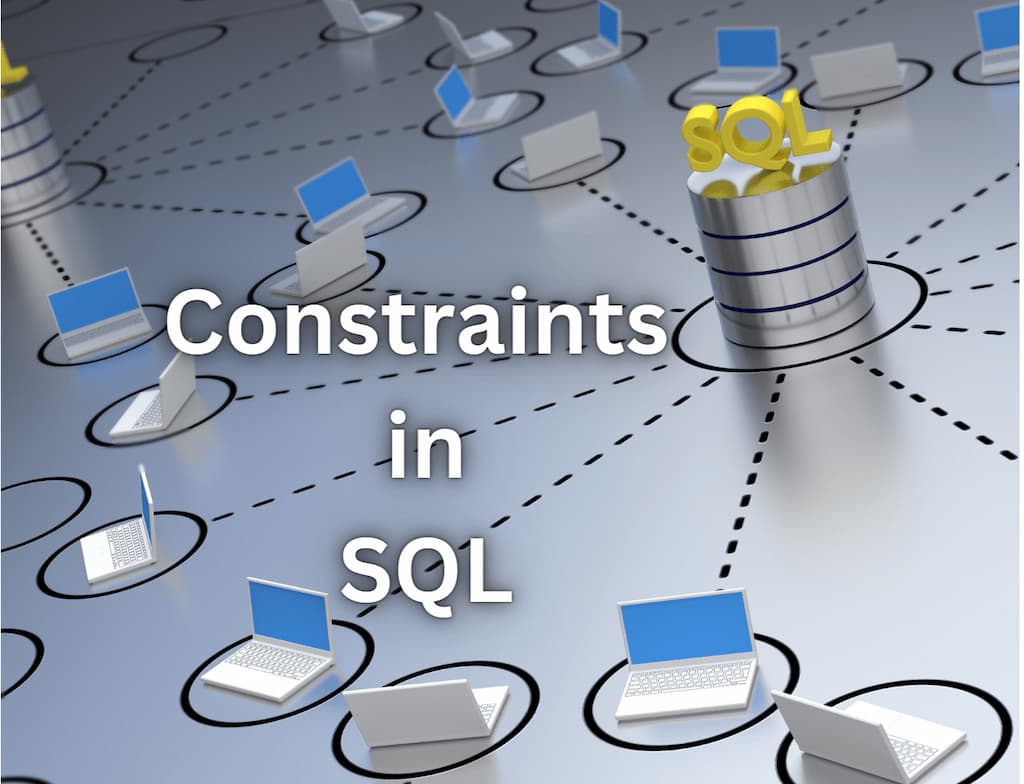
Explore the various MySQL constraints, their definitions, and practical examples to maintain data integrity and consistency in your databases.
The Complete Guide to MySQL Constraints: A Comprehensive Explanation
MySQLconstraintsare essential rules enforced on database columns to maintaindata integrity, accuracy, and consistency. Constraints prevent invalid data from entering the database and ensure relationships between tables are correctly maintained.
This guide coversall MySQL constraints, including theirsyntax, variations (single-column, multi-column, and dependent constraints), and real-world examples.
Aconstraintin MySQL is a rule applied to a column or table to restrict the values that can be stored. Constraints ensure that only valid and meaningful data is stored in a database.
NULL
values.Before demonstrating constraints, let'screate a sample database and tables.
CREATE DATABASE CompanyDB;
USE CompanyDB;
CREATE TABLE Employees (
employee_id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
email VARCHAR(100) UNIQUE,
age INT CHECK (age >= 18 AND age <= 65),
salary DECIMAL(10,2) DEFAULT 3000.00,
department_id INT,
FOREIGN KEY (department_id) REFERENCES Departments(department_id)
);
Ensures that a columncannot have NULL
values.
CREATE TABLE Employees (
employee_id INT PRIMARY KEY,
name VARCHAR(50) NOT NULL
);
name
cannot be NULL
.
CREATE TABLE Employees (
employee_id INT NOT NULL,
name VARCHAR(50) NOT NULL
);
Both employee_id
and name
must have values.
Ensures that all values in a columnare distinct.
CREATE TABLE Employees (
email VARCHAR(100) UNIQUE
);
email
values must be unique.
CREATE TABLE Employees (
first_name VARCHAR(50),
last_name VARCHAR(50),
UNIQUE (first_name, last_name)
);
Ensures no two employees have the same first_name
and last_name
.
APRIMARY KEYuniquely identifies each record andcannot be NULL
.
CREATE TABLE Employees (
employee_id INT PRIMARY KEY
);
Each employee_id
must be unique and not NULL
.
CREATE TABLE EmployeeProjects (
employee_id INT,
project_id INT,
PRIMARY KEY (employee_id, project_id)
);
A composite key ensures uniqueness across multiple columns.
AFOREIGN KEYensures referential integrity by linking a column to another table’s primary key.
CREATE TABLE Employees (
employee_id INT PRIMARY KEY,
department_id INT,
FOREIGN KEY (department_id) REFERENCES Departments(department_id)
);
department_id
in Employees
must exist in Departments
.
CREATE TABLE Orders (
order_id INT,
product_id INT,
customer_id INT,
PRIMARY KEY (order_id, product_id),
FOREIGN KEY (customer_id, product_id) REFERENCES Customers(customer_id, product_id)
);
Ensures that the combination of customer_id
and product_id
exists in the Customers
table.
Ensures a columnsatisfies a condition.
CREATE TABLE Employees (
age INT CHECK (age >= 18 AND age <= 65)
);
age
must be between 18 and 65.
CREATE TABLE Employees (
salary DECIMAL(10,2),
bonus DECIMAL(10,2),
CHECK (salary >= bonus * 2)
);
Ensures salary
is at least twice the bonus
.
Assigns adefault valueto a column.
CREATE TABLE Employees (
salary DECIMAL(10,2) DEFAULT 3000.00
);
If salary
is not provided, it defaults to 3000.00.
Automatically generates auniquenumeric value for each row.
CREATE TABLE Employees (
employee_id INT PRIMARY KEY AUTO_INCREMENT
);
employee_id
auto-increments with each new record.
Summary of MySQL Constraints
Constraint | Purpose |
---|---|
NOT NULL | Prevents NULL values |
UNIQUE | Ensures column values are unique |
PRIMARY KEY | Uniquely identifies records |
FOREIGN KEY | Enforces referential integrity |
CHECK | Validates data based on a condition |
DEFAULT | Assigns a default value if none provided |
AUTO_INCREMENT | Auto-generates unique numbers |
INDEX | Improves search performance |
Conclusion
MySQL constraints ensuredata integrity, accuracy, and consistency. Understanding how to use constraints properly is crucial in designing robust databases.
Master these constraints to build high-quality, error-free MySQL databases!