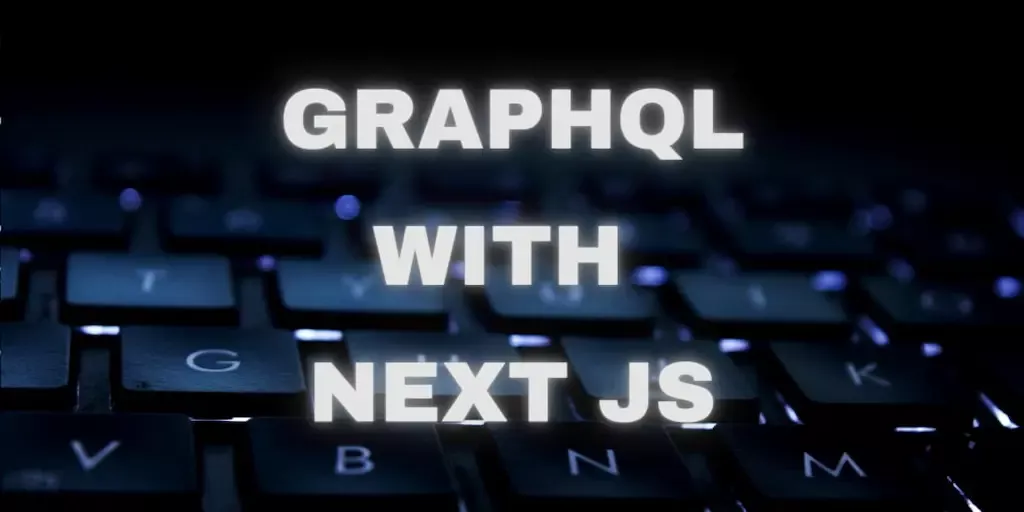
Hello Everyone Today i will be discussing GraphQL API with Next JS. GraphQL is an open-source query...
Hello Everyone Today i will be discussing GraphQL API with Next JS.
GraphQL is an open-source query language and runtime for APIs (Application Programming Interfaces). It provides a flexible and efficient approach for clients to request and manipulate data from a server. GraphQL was developed by Facebook and released to the public in 2015.
Unlike traditional REST APIs, where clients often receive fixed data structures determined by the server, GraphQL allows clients to define the shape and structure of the data they need. Clients can send a single request to the server, specifying the exact data requirements, and receive a response that matches the requested structure. This eliminates the problem of over-fetching or under-fetching data, where clients receive more or less data than they actually need.
Here's a step-by-step guide to performing CRUD operations with GraphWL, Express JS, MongoDB, and NEXT JS -
- project/
- components/
- Books.js
- pages/
- index.js
- apolloClient.js
- server.js
- package.json
We will Create a Simple Book Storing APP for Adding, updating, fetching, and deleting a book.
npx create-next-app graphql-next-app
Go into that directory
cd graphql-next-app
npm install express mongoose next apollo-server-express graphql apollo-client apollo-link-http graphql
const express = require('express');
const { ApolloServer, gql } = require('apollo-server-express');
const mongoose = require('mongoose');
// Connect to MongoDB
mongoose.connect('mongodb://localhost/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true });
const app = express();
const typeDefs = gql`
type Book {
id: ID!
title: String!
author: String!
}
type Query {
books: [Book]
book(id: ID!): Book
}
type Mutation {
createBook(title: String!, author: String!): Book
updateBook(id: ID!, title: String, author: String): Book
deleteBook(id: ID!): Boolean
}
`;
const books = [
{ id: '1', title: 'Book 1', author: 'Author 1' },
{ id: '2', title: 'Book 2', author: 'Author 2' },
];
const resolvers = {
Query: {
books: () => books,
book: (parent, { id }) => books.find((book) => book.id === id),
},
Mutation: {
createBook: (parent, { title, author }) => {
const newBook = { id: String(books.length + 1), title, author };
books.push(newBook);
return newBook;
},
updateBook: (parent, { id, title, author }) => {
const book = books.find((book) => book.id === id);
if (!book) {
throw new Error('Book not found');
}
book.title = title || book.title;
book.author = author || book.author;
return book;
},
deleteBook: (parent, { id }) => {
const bookIndex = books.findIndex((book) => book.id === id);
if (bookIndex === -1) {
throw new Error('Book not found');
}
books.splice(bookIndex, 1);
return true;
},
},
};
const server = new ApolloServer({ typeDefs, resolvers });
server.applyMiddleware({ app });
app.listen({ port: 4000 }, () =>
console.log(`Server ready at http://localhost:4000${server.graphqlPath}`)
);
Run the following command to start the server
node server.js
import { ApolloClient, InMemoryCache, HttpLink } from '@apollo/client';
const client = new ApolloClient({
link: new HttpLink({
uri: 'http://localhost:4000/graphql', // Update with your server URL
}),
cache: new InMemoryCache(),
});
export default client;
import { useQuery, useMutation, gql } from '@apollo/client';
const GET_BOOKS = gql`
query {
books {
id
title
author
}
}
`;
const CREATE_BOOK = gql`
mutation CreateBook($title: String!, $author: String!) {
createBook(title: $title, author: $author) {
id
title
author
}
}
`;
const UPDATE_BOOK = gql`
mutation UpdateBook($id: ID!, $title: String, $author: String) {
updateBook(id: $id, title: $title, author: $author) {
id
title
author
}
}
`;
const DELETE_BOOK = gql`
mutation DeleteBook($id: ID!) {
deleteBook(id: $id)
}
`;
const Books = () => {
const { loading, error, data } = useQuery(GET_BOOKS);
const [createBook] = useMutation(CREATE_BOOK);
const [updateBook] = useMutation(UPDATE_BOOK);
const [deleteBook] = useMutation(DELETE_BOOK);
const handleCreateBook = async () => {
try {
const { data } = await createBook({ variables: { title: 'New Book', author: 'New Author' } });
console.log('New book created:', data.createBook);
} catch (error) {
console.error('Error creating book:', error);
}
};
const handleUpdateBook = async (id, title, author) => {
try {
const { data } = await updateBook({ variables: { id, title, author } });
console.log('Book updated:', data.updateBook);
} catch (error) {
console.error('Error updating book:', error);
}
};
const handleDeleteBook = async (id) => {
try {
const { data } = await deleteBook({ variables: { id } });
console.log('Book deleted:', data.deleteBook);
} catch (error) {
console.error('Error deleting book:', error);
}
};
if (loading) {
return <p>Loading...</p>
}
if (error) {
returnError: {error.message};
}
return (
<div><button onclick="{handleCreateBook}">Create Book</button>
<ul>{data.books.map((book) => (
<li key="{book.id}">{book.title} by {book.author}{' '} <button onclick="{()"> handleUpdateBook(book.id, 'Updated Title', 'Updated Author')}> Update </button>{' '} <button onclick="{()"> handleDeleteBook(book.id)}>Delete</button></li>
))}</ul>
</div>
);
};
export default Books;
Open the pages/index.js file and replace its contents with the following code:
import Books from '../components/Books';
const Home = () => {
return (
<div>
<h1>Books</h1>
<books> </books></div>
);
};
export default Home;
npm run dev
Congratulations you have created a basic Full Stack app with GraphQL, Express JS, MongoDB, and Next JS.