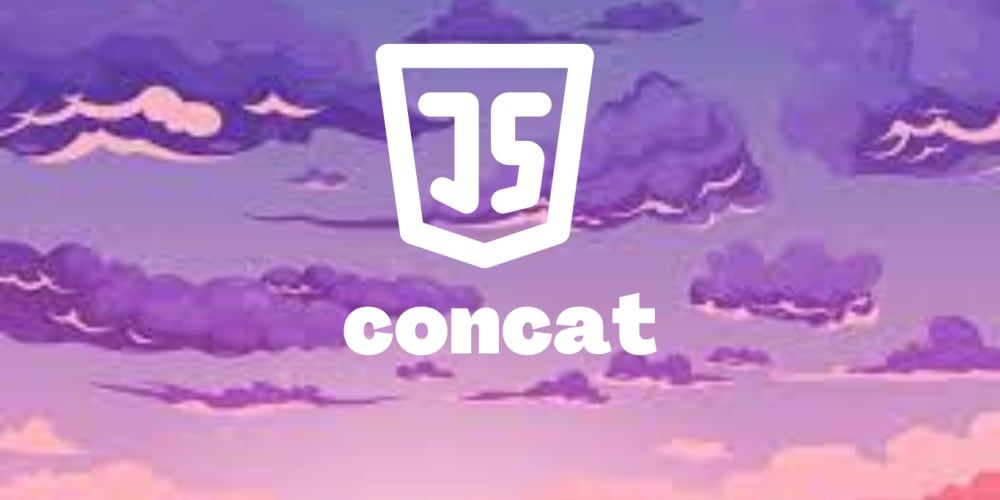
Hello guys today i will show you how to use inbuilt concat array method in javascript. What...
Concat is used to merge two or more arrays and it always returns a new array instead of modifying the original arrays.
const array1 = [1,2,3,4,5,6];
const array2 = ["A","B","C","D"];
const array1ToArray2 = array1.concat(array2)
const array2ToArray1 = array2.concat(array1)
console.log(array1ToArray2)
console.log(array2ToArray1)
[
1, 2, 3, 4, 5,
6, 'A', 'B', 'C', 'D'
]
[
'A', 'B', 'C', 'D', 1,
2, 3, 4, 5, 6
]
const array1 = [1,2,3,4,5,6];
const array2d = [[1,2],[3,4],[[5,6],[7,8]]];
const array1To2dArray = array1.concat(array2d)
console.log(array1To2dArray);
[ 1, 2, 3, 4, 5, 6, [ 1, 2 ], [ 3, 4 ], [ [ 5, 6 ], [ 7, 8 ] ] ]
const array1 = [1,2,3,4,5,6];
const separateConcatening = array1.concat(7,8,9,"E","F",true,false,null,undefined,
[10,11,"G","H"],{name:"shubham",age:21},12.967)
console.log(separateConcatening)
[1,2,3,4,5,6,7,8,9,'E','F',true,false,null,undefined,
10,11,'G','H',{ name: 'shubham', age: 21 },12.967
]
const array1 = [1,2,3,4,5,6];
const array2 = ["A","B","C","D"];
const array2d = [[1,2],[3,4],[[5,6],[7,8]]];
const concatArray = (...args) => {
return args.flat(Infinity)
}
console.log(concatArray([1,2,3,4],[5,6,7,8],array1,array2,array2d))
[
1, 2, 3, 4, 5, 6, 7, 8,
1, 2, 3, 4, 5, 6, 'A', 'B',
'C', 'D', 1, 2, 3, 4, 5, 6,
7, 8
]
Example - 5
const array1 = [1,2,3,4,5,6];
const array1ToArray1 = array1.concat(array1)
console.log(array1ToArray1)
[
1, 2, 3, 4, 5,
6, 1, 2, 3, 4,
5, 6
]
const array1 = [1,2,3,4,5,6];
const array2 = ["A","B","C","D"];
const array2d = [[1,2],[3,4],[[5,6],[7,8]]];
const array1ToMultiple = array1.concat(array1,array2,array2d)
console.log(array1ToMultiple)
[1,2,3,4,5,6,1,2,3,4,5,6,'A', 'B', 'C', 'D',[ 1, 2 ],
[ 3, 4 ],
[ [ 5, 6 ], [ 7, 8 ] ]]
We can also merge multiple arrays by passing them inside concat method.