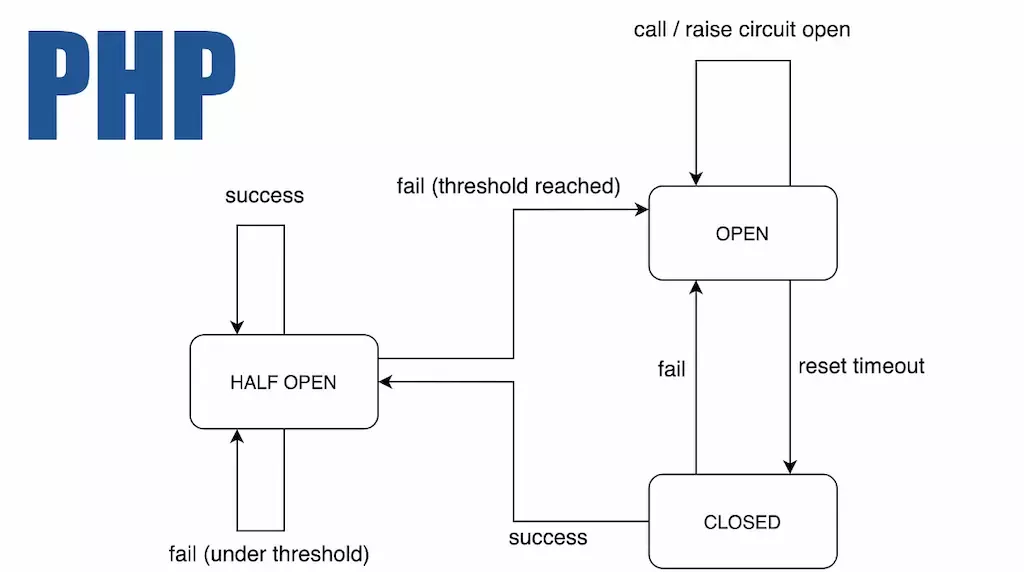
The Circuit Breaker pattern is a design pattern used in software development to enhance the stability and resilience of a system
The Circuit Breaker pattern is a design pattern used in software development to enhance the stability and resilience of a system by preventing it from repeatedly making calls to a service or component that is likely to fail. This pattern is particularly useful in distributed systems and microservices architectures. It helps to protect your application from cascading failures due to repeatedly calling a failing service.
The Circuit Breaker pattern is a powerful tool for improving the resilience and stability of distributed systems and microservices architectures. It’s especially useful when dealing with services or components that are prone to failures. Let’s explore some common use cases, pros, and cons of the Circuit Breaker pattern.
Use Cases:
Pros:
Cons:
In PHP, you can implement the Circuit Breaker pattern using a combination of classes and strategies. Here’s a simplified example of how you could implement a Circuit Breaker pattern in PHP:
class CircuitBreaker {
private $state = 'closed';
private $failureThreshold = 3;
private $resetTimeout = 300;// 5 minutes in seconds
private $failureCount = 0;
private $lastFailureTime = null;
public function execute(callable $operation) {
if ($this->state === 'open' && $this->isTimeoutReached()) {
$this->state = 'half-open';
}
if ($this->state === 'closed' || $this->state === 'half-open') {
try {
$result = $operation();
$this->reset();
return $result;
} catch (Exception $e) {
$this->handleFailure();
throw $e;
}
}
throw new CircuitBreakerOpenException("Circuit breaker is open");
}
private function handleFailure() {
$this->failureCount++;
$this->lastFailureTime = time();
if ($this->failureCount >= $this->failureThreshold) {
$this->state = 'open';
}
}
private function isTimeoutReached() {
return time() - $this->lastFailureTime >= $this->resetTimeout;
}
private function reset() {
$this->failureCount = 0;
$this->lastFailureTime = null;
$this->state = 'closed';
}
}
class CircuitBreakerOpenException extends Exception {}
// Usage
$circuitBreaker = new CircuitBreaker();
try {
$result = $circuitBreaker->execute(function () {
// Call the service or perform an operation here
// For example, making an HTTP request or accessing a resource
// return $response;
});
echo $result;
} catch (CircuitBreakerOpenException $e) {
echo "Circuit breaker is open, please try again later.";
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
In this example, the CircuitBreaker
class
manages the state of the circuit breaker and determines whether to allow an operation to proceed or throw an
exception based on the circuit's state. The circuit can be in one of three states: "closed," "open," or "half-open."
Please note that this example provides a simplified implementation of the Circuit Breaker pattern. In a real-world scenario, you might need to handle more complex situations and integrate the pattern into your application’s architecture.
In summary, the Circuit Breaker pattern is a valuable tool for enhancing system reliability and stability, especially in distributed and microservices architectures. It offers significant benefits in preventing cascading failures and improving overall application resilience. However, it should be used judiciously and configured carefully to avoid false positives and other potential drawbacks.