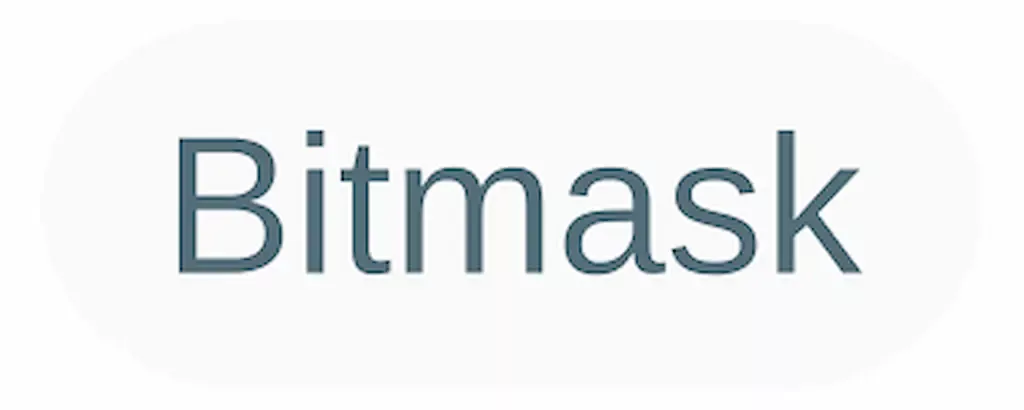
Bitmasks are as old as computing itself and, admittedly, were more useful in the days of memory scarcity and low-level programming. But there’s nothing to stop you using them today, when appropriate.
A bitmask is a technique used in computer science and programming to represent a collection of binary values or flags compactly using a sequence of bits. Each individual bit in the sequence corresponds to a specific option, attribute, or flag. Bitmasks are commonly used for various purposes, including representing and manipulating sets of options or attributes.
In a bitmask, each bit can be thought of as a switch that can be turned on (1) or off (0). By assigning specific meanings to each bit position, you can represent different options or states in a concise manner. Bitwise operations are then used to manipulate and query the individual bits of the bitmask.
Bitmasks are particularly useful for scenarios where multiple binary options need to be tracked efficiently within a single value. They are often used in areas such as:
For example, consider a scenario where you have three options: A, B, and C. Each option can be either enabled (1) or disabled (0). You can represent these options using a 3-bit bitmask:
000
represents no options enabled.001
represents option A enabled.010
represents option B enabled.011
represents options A and B enabled.100
represents option C enabled.101
represents options A and C enabled.110
represents options B and C enabled.111
represents all options (A, B, and C) enabled.Bitmasks provide an efficient way to store and manipulate multiple binary states, often using a single integer or a sequence of bits. However, they require a solid understanding of bitwise operations to work with effectively.
Here’s how you can work with bitmasks in PHP:
Setting Bits:
You can set specific bits in a bitmask using the bitwise OR (|
) operator.
$bitmask = 0; // Initialize bitmask
$bitmask |= 1; // Set first bit (2^0)
$bitmask |= 4; // Set third bit (2^2)
$bitmask |= 16; // Set fifth bit (2^4)
Checking Bits:
You can check if a specific bit is set in a bitmask using the bitwise AND (&
) operator.
$bitmask = 21; // Assume this is your bitmask
if ($bitmask & 1) {
echo "First bit is set.\n";
}
if ($bitmask & 4) {
echo "Third bit is set.\n";
}
Clearing Bits:
You can clear specific bits in a bitmask using bitwise NOT (~
) and bitwise AND (&
) operators.
$bitmask = 21; // Assume this is your bitmask
$bitmask &= ~4; // Clear the third bit (2^2)
Toggling Bits:
You can toggle specific bits in a bitmask using the bitwise XOR (^
) operator.
$bitmask = 21; // Assume this is your bitmask
$bitmask ^= 16; // Toggle the fifth bit (2^4)
Bitmasks are often used to represent sets of options or flags compactly, such as user permissions, feature enable/disable states, or any scenario where multiple binary options need to be tracked efficiently within a single value.
Keep in mind that while bitmasks can be powerful and memory-efficient, they require careful handling and understanding of bitwise operations. It’s also important to use meaningful constant names for your bit positions to make your code more readable and maintainable.