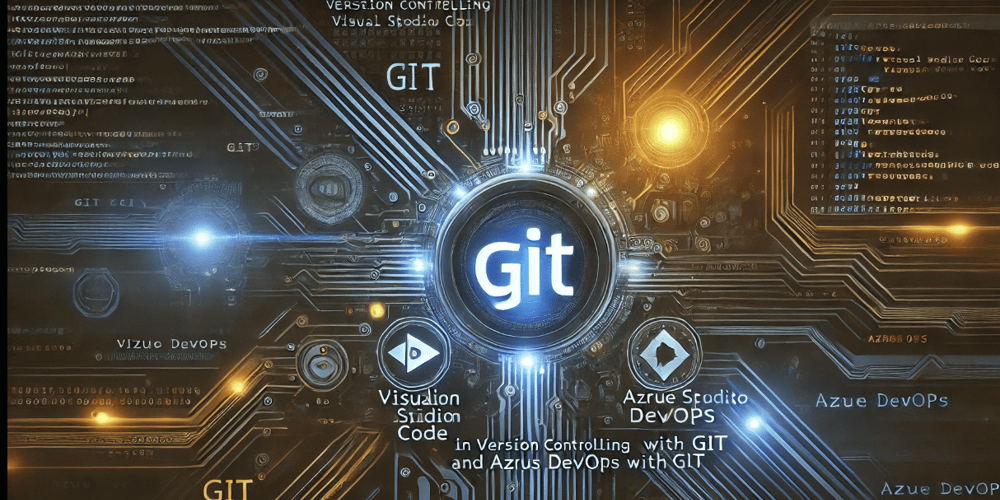
In this exercise, we will be learning the foundations of version control using Git on Azure...
Git is adistributed version control system (DVCS)that helps developers track changes in their code, collaborate efficiently, and manage project history. It enables teams to work on the same project simultaneously, merge changes, and revert to previous versions when needed.
Git Repository:
AGit repository (repo)is a storage space for your project's code, including its entire history of changes. Repositories can be:
Branching in Git
Each branch in Git can have both alocalandremoteversion.
To share changes:
1. Commit Locally– First, commit the changes on your local machine (on the local branch).
2. Push to Remote– Push the committed changes to the remote version of that branch.
3. Merge to Main– Once the changes are tested and ready, they can be merged from the remote branch into the main
branch.
CI/CD (Continuous Integration/Continuous Deployment):
CI/CDis a software development practice that automates testing, building, and deploying code. It helps ensure fast and reliable software delivery and updates by:
Azure DevOps is a cloud-based platform that providesGit repositories, CI/CD pipelines, and project management tools. It allows teams to store, manage, and collaborate on code efficiently.
In your Azure portal, search for and select Azure Dev Ops Organizations
Click My Azure DevOps Organizations
Click "create new organization"
Click continue, name your organization (must be globally unique), select region to host, and enter the security characters
Click continue again. Your organization will now be created
Since this organization is new, we need to enable the creation of classic build and release pipelines
In the Azure DevOps portal, select your newly created organization
Select Organization Settings
Under Pipelines, select Settings
Make sure these settings are disabled:
Now we need to configure the Parts Unlimited team project. This is needed for certain Azure DevOps labs. In this case, we will use it as a sample codebase to practice Git operations on, simulating real use of the Azure DevOps platform and Git commands.
Go to https://azuredevopsdemogenerator.azurewebsites.net
This site will automate the process of creating a new Azure DevOps project within your account that is prepopulated with content (work items, repos, etc.) required for the lab.
Click Sign in and enter your Azure Dev Ops account information
Read and Accept the permissions
Click choose template and select Parts Unlimited
Type parts Unlimited as the Project Name
Select the organization previously created and click create project
Click "Navigate to project"
If you're redirected to 404 page, saying project doesn't exist, follow these steps in organization settings:
Once these settings are changed, create the project again following the previous steps
In this task, we will configure aGit credential helperto securely store the Git credentials used to communicate withAzure DevOps.
git config --global credential.helper wincred
git config --global user.name "Your name"
git config --global user.email youremail@example.com
Cloning a repository means creating a local copy of a remote Git repository on your machine. This allows you to work on the project locally, make changes, and sync with the remote repository when needed.
When you clone a repository, Git downloads all the files, history, and branches, allowing you to contribute or experiment without affecting the original remote repository.
Navigate to Repos hub
Click clone
Copy the clone URl
In visual studio code, pressCtrl+Shift+Pon Windows orCMD+Shift+Pto show the command Palete
Search for and select Git Clone
Paste the project clone URL that you copied
Paste the project clone URL that you copiedGo back to your browser where you have the repository open. Click "Generate Git credentials"
Store the user name and password. Copy the password
Enter the password when prompted in the command pallete
You will have to specify or create a destination folder for the project to be cloned into
Open repository
On Vs code, with the repository open, select command + p (mac) or control + p (windows) to search for files. Search for and select Cartitems.cs
Create a comment and write anything. This can be done by entering '//' before your comment message. We are simulating a change in the code here. Save the file (command / control + s).
Commiting your code saves the state of changes to your local machine on your local branch.
Run the following commands in the terminal:git status
Explanation:
git status
git add .
git commit -m "Added a sample comment to simulate a change"
Explanation:
git add .
This command stages all the changes in your working directory, preparing them for commit.
git commit -m "Your message"
This command saves the changes with a commit message, allowing you to track what was modified.
The commands should have a result like this:
Once your changes are committed locally, you need topushthem to the remote repository so that others can access them.
git remote -v
You should see something like:
origin https://github.com/your-username/your-repository.git (fetch)
origin https://github.com/your-username/your-repository.git (push)
git push
Check the repo in Azure you Azure DevOps account. Under Repos > Commits, you should see the latest push.
If you see a failure message like in the picture above, you may need to fill out the form here
Staging specific changes allows you to choose what and what not to commit. In the last example, we commited all changes by using the command git add
.
We will now modify two different files but we will only commit and push the changes in one of these two files,
In VS code, open the CartItems.cs
file again. Add another comment like so, Save the file:
Press command + p
or control + p
(Mac and Windows Respectively) and search for and select the Category.cs
file
Make a new comment. Ex: 'comment that wont be committed', Save the file
In the terminal, enter:
git status
you should see that no changes are currently staged
Then enter:
git add PartsUnlimited-aspnet45/src/PartsUnlimitedWebsite/Models/CartItem.cs
CartItem.cs
git commit -m "updated cartitems"
This will commit the changes from only CartItem.cs
to your local branch
Finally, enter:
git push
This will push the changes to the remote branch. Only CartItems.cs
will be updated remotely.
In the Azure DevOps portal, navigate to Repos > commits and you will see the latest commit
You will notice that only the CartItems.cs was updated
A comparison view will be opened to enable you to easily locate the changes you’ve made. In this case, it’s just the one comment.
In Azure DevOps portal, in the commits view, you can see the latest commit. By clicking the 3 dot menu, you can select browse files to see the changes in each commit
Branches allow you to develop features, fix bugs, or experiment without affecting the main codebase. These changes can then be merged into the main codebase when properly tested
To see all branches in your repository, run:
git branch -a
Currently, we you be on a master branch. Press q
to exit branches view
git checkout -b dev
This will create a new branch called dev
The current branch (master) will be used as the starting point for this new branch. In the terminal, you will see that you are now on the dev
branch
dev
. There is no corresponding remote version of the dev
branch. To publish a remote version of this branch in Azure DevOps, enter: git push --set-upstream origin dev
In the branches view, hover over the dev branch, select the 3 dot menu, and click delete branch. Confirm the deletion
The remote dev branch is now deleted. However, the local one on your machine still exists. To delete it, first go to the terminal
Enter this command to switch to the master
branch (we cannot delete the dev branch if we are currently on it)
git checkout master
dev
branchgit branch -d dev
git fetch --prune
Call this branch release. It will be based on the master branch. Click create
The branch should appear in the list
You must now update your local git environment with the proper branch information. To do this type this command int he terminal:
git fetch
release
branch locally by enteringgit checkout release
Restoring a remote branch
Delete the release branch. Confirm the delete when prompted
Type release in the query labeled search branch name
Hover over the release branch, over the 3 dots, then select restore branch
The remote branch is now restored
In the Repos > Branches view, hover over the 3 dot menu on the master branch. Select Lock
Unlock with the same process
Areleasein Git represents a specific version of your project at a given point in time. It is typically used for:
Releases are often associated withtagsin Git, which serve as reference points for specific commits.
Repos
, select Tags
New Tag
Enter a description, then click create
You have now tagged the project at this release. You can tag commits for a variety of reasons. Azure DevOps offers the flexibility to edit and delete them, as well as manage their permissions.
From the project Add dropdown, select New repository.
Pick a new Repository name. Notice that you also have the option to create a file named README.md
. This would be the default markdown file that is rendered when someone navigates to the repo root in a browser. It usually contains general information about the code base/project. You can also initialize the repo with a .gitignore
file. This file specifies which files, based on naming pattern and/or path, that will not be tracked and added into the Repository. This is useful for secret files that contain private information and need to stay on your local machine. There are several templates available that include the common patterns and paths to ignore based on the project type you are creating. Click Create.
Select the Repository recently made
Notice the rename and the delete options
In this guide, we explored the fundamentals ofGit version controlinVisual Studio CodeandAzure DevOps.
git commit
.git status
to check modifications before committing.git checkout -b
, allowing for isolated development.git push
.