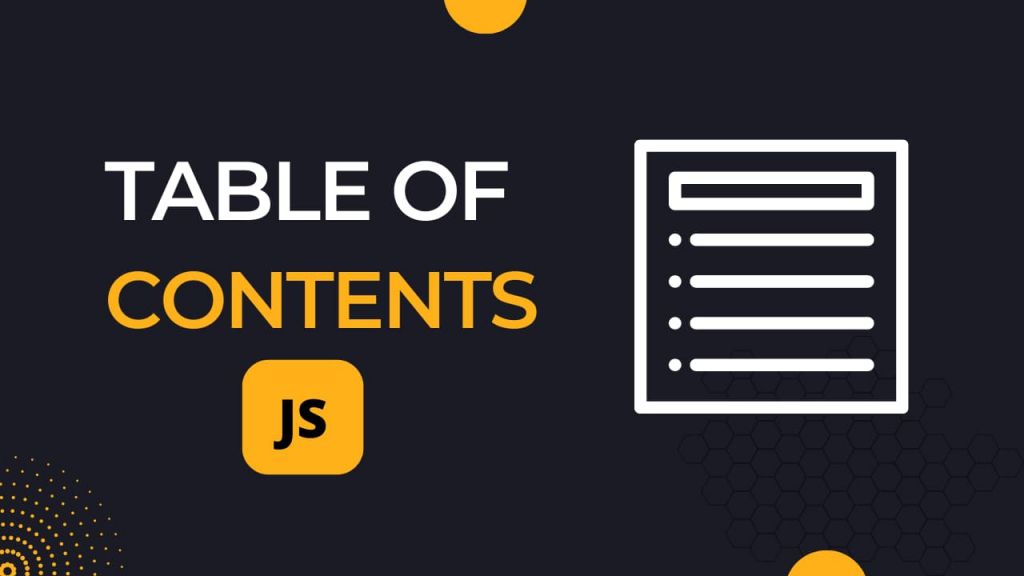
A quick tutorial for beginners, how to show a (flat) Javascript array in a "nice HTML table". ...
A quick tutorial for beginners, how to show a (flat) Javascript array in a "nice HTML table".
<!-- (A) EMPTY TABLE -->
<table id="demo"></table>
<script>
// (B) ARRAY OF DATA
var data = ["Alpaca", "Birb", "Cate", "Doge", "Eagle", "Foxe"];
// (C) CREATE TABLE ROWS & CELLS
var table = "<tr>",
perrow = 2, cells = 0;
data.forEach((value, i) => {
// (C1) ADD CELL
table += `<td>${value}</td>`;
// (C2) CLICK ON CELL TO DO SOMETHING
// table += `<td onclick="alert($)">${value}</td>`;
// (C3) BREAK INTO NEXT ROW
cells++;
if (cells%perrow==0 && cells!=data.length) { table += "</tr><tr>"; }
});
table += "</tr>";
// (D) ATTACH TO EMPTY TABLE
document.getElementById("demo").innerHTML = table;
</script>
<table>
.var table = "<tr>";
data.forEach((value, i) => { table += `<td>$</td>`; };
perrow = 2
cells
flag to track the current cell.cells%perrow==0
cells!=data.length
<!-- (A) EMPTY TABLE -->
<table id="demo"></table>
<script>
// (B) ARRAY OF DATA
var data = ["Alpaca", "Birb", "Cate", "Doge", "Eagle", "Foxe"];
// (C) CREATE TABLE ROWS & CELLS
var table = document.getElementById("demo"),
row = table.insertRow(), cell,
perrow = 2, cells = 0;
data.forEach((value, i) => {
// (C1) ADD CELL
cell = row.insertCell();
cell.innerHTML = value;
// (C2) CLICK ON CELL TO DO SOMETHING
cell.onclick = () => alert(i);
// (C3) BREAK INTO NEXT ROW
cells++;
if (cells%perrow==0 && cells!=data.length) { row = table.insertRow(); }
});
table = document.getElementById("demo");
.row = table.insertRow();
cell = row.insertCell(); cell.innerHTML = value;